Avoid form resubmission on page refresh using PHP or JavaScript.
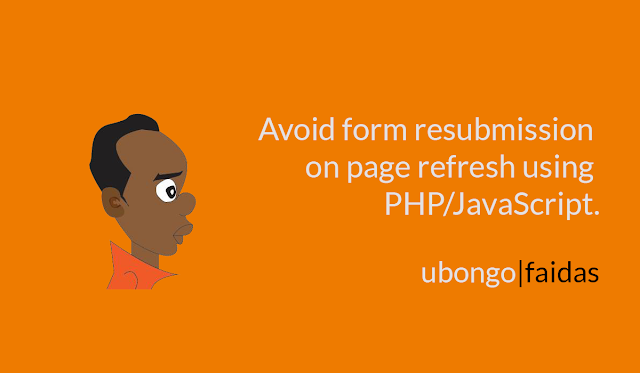
When you submit data to a database via an HTML form, those data are inserted into the database, but if you click the submit button again without entering any data into the input field you will find the same data stored in the database, again when you try to refresh the page, you will find the same data are inserted. In that sense you will have duplicated data in your database, thus causing trouble when updating or deleting data.
There are three ways to prevent this problem, You can choose the best method that suits your project.
Using the PHP redirect function
If there is no need for the user to remain on the same page after submitting data, then a good way to avoid form resubmission on page refresh is a PHP redirect function that can redirect the user to another page automatically.
PHP redirect function is written this way
header('Location: destination_page.php');
so you can replace "destination_page.php" with the page you want to redirect the user to. Check out the full example below;
<?php if(isset($_POST['comment'])){ //validation code for variable $comment_body, $member_id goes here $comment_body = $_POST['comment_body']; $member_id = $_POST['member_id']; if (count($errors) === 0){ $insert= $connect->prepare("INSERT INTO comment (comment_body, member_Id) VALUES (?, ?)"); $insert->execute([$comment_body, $member_id]); //now redirect user to another page header('location: destination_page.php'); } } ?>
Using the unset function
The unset function is another way that can be used to avoid form resubmission on page refresh. All you have to do is set a variable that carries the data and puts it in parentheses as a parameter.
The unset function is written in this way:
unset($variable); //or unset $variable;
Check out the example code here;
<?php if(isset($_POST['comment'])){ //validation code for variable $comment_body, $member_id goes here $comment_body = $_POST['comment_body']; $member_id = $_POST['member_id']; if (count($errors) === 0){ $insert= $connect->prepare("INSERT INTO comment (comment_body, member_Id) VALUES (?, ?)"); $insert->execute([$comment_body, $member_id]); //now use unset function unset($insert); } } ?>
Using Javascript history.replaceState()
history.replaceState() replaces a single entry in the browser session history. This means that the data you submit, will not be stored on your browser as soon as you send it, so you will have to send it again because the browser will not remember the first time it was there and this can avoid the form from resubmitting data on-page refresh. If you want to understand the syntax click here.
Now you'll need to create an external Javascript file or go directly with internal coding style, then include the following between body tags in an HTML document.
<script type="text/javasricpt"> if(window.history.replaceState ) { window.history.replaceState( null, null, window.location.href ); } </script>
0 Comments